AudioBuffer Class¶
Class for storing and passing audio signals around. Can be instantiated with an array of audio samples or location to load audio from disk. Stores sample rate, number of channels, and the file name if loaded from disk.
Also has utility functions for loading and saving audio from disk, normalizing, resizing buffers, and plotting spectrograms.
Examples
Create a new empty AudioBuffer
:
>>> audio = spiegelib.AudioBuffer()
Create an AudioBuffer
from existing audio samples:
>>> audio = spiegelib.AudioBuffer(audio_samples, 44100)
Load audio from disk into a new AudioBuffer
:
>>> audio = spiegelib.AudioBuffer('./some_audio_file.wav')
Load an entire folder of audio samples into a list of AudioBuffer
objects:
>>> audio_files = spiegelib.AudioBuffer.load_folder('./audio_folder')
Plot a spectrogram
1 2 3 4 5 6 | import spiegelib as spgl
import matplotlib.pyplot as plt
audio = spgl.AudioBuffer('./audio_file.wav')
audio.plot_spectrogram()
plt.show()
|
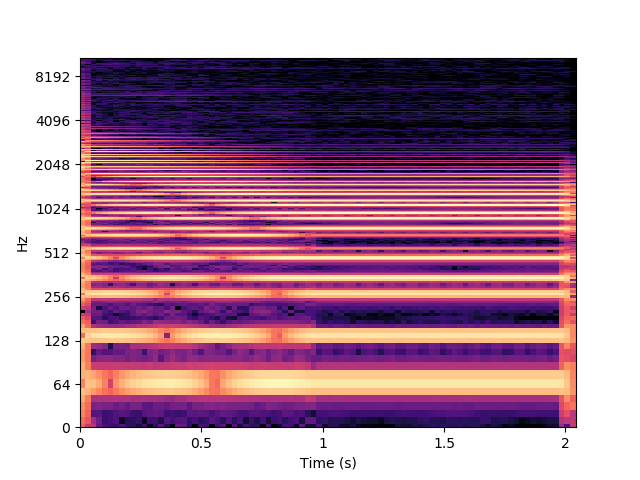
-
class
spiegelib.
AudioBuffer
(input=None, sample_rate=None, **kwargs)¶ - Parameters
input (np.ndarray, list, str, file-like object) – Can be an array of audio samples (np.ndarray or list), or a path to a location of an audio file on disk. Defaults to None.
sample_rate (int) – Rate of sampled audio if audio data was passed in, or rate to resample audio data loaded from disk at, defaults to None. If loading audio from a file, will automatically resample to 44100 if no sample rate provided.
kwargs – keyword args to pass into librosa load function.
-
get_audio
()¶ Getter for audio data
- Returns
Array of audio samples
- Return type
np.ndarray
-
get_sample_rate
()¶ Getter for sample rate
- Returns
Sample rate of audio buffer
- Return type
int
-
load
(path, sample_rate=44100, **kwargs)¶ Read audio from a file into a numpy array at specific audio rate. Stores in this
AudioBuffer
object.- Parameters
path (str, int, pathlib.Path, or file-like object) – location of audio file on disk
sample_rate (int) – resample audio to this rate, defaults to 44100. None uses native sampling rate.
kwargs –
keyword args to pass into librosa load function.
-
plot_spectrogram
(**kwargs)¶ Plot spectrogram of this audio buffer. Uses librosas specshow function. Uses matplotlib.pyplot to plot spectrogram.
Defaults to logarithmic y-axis in Hertz and seconds along the x-axis.
- Parameters
kwargs –
see specshow.
- Returns
The axis handle for figures
- Return type
axes
-
replace_audio_data
(audio, sample_rate)¶ Replace audio data in this object
- Parameters
audio (np.ndarray) – Array of audio samples
sample_rate (int) – Rate that audio data was sampled at
-
resize
(num_samples, start=0)¶ Resize audio to a set number of samples. If new length is less than the current audio buffer size, then the buffer will be trimmed. If the new length is greater than current audio buffer, then the resulting buffer will be zero-padded at the end.
- Parameters
num_samples (int) – New length of audio buffer in samples
start_sample (int) – Start reading from certain number of samples into current buffer
-
save
(path, normalize=False)¶ Save an audio to disk as WAV file at given sample rate. Can normalize before saving as well.
- Parameters
path (str or open file handle) – location to save audio to
normalize (boolean) – normalize audio before writing, defaults to false
-
static
peak_normalize
(audio)¶ Peak normalize audio data
- Parameters
audio (np.ndarray) – array of audio samples
- Returns
normalized array of audio samples
- Return type
np.ndarray
-
static
load_folder
(path, sort=True, sample_rate=44100, **kwargs)¶ Try to load a folder of audio samples
- Parameters
path (str) – Path to directory of audio files
sort (bool) – Apply natural sort to file names. Default True
sample_rate (int) – Sample rate to load audio files at. Defualts to 44100
kwargs –
keyword args to pass into librosa load function.
- Returns
List of
AudioBuffer
objects- Return type
list